Git Branching Workflow
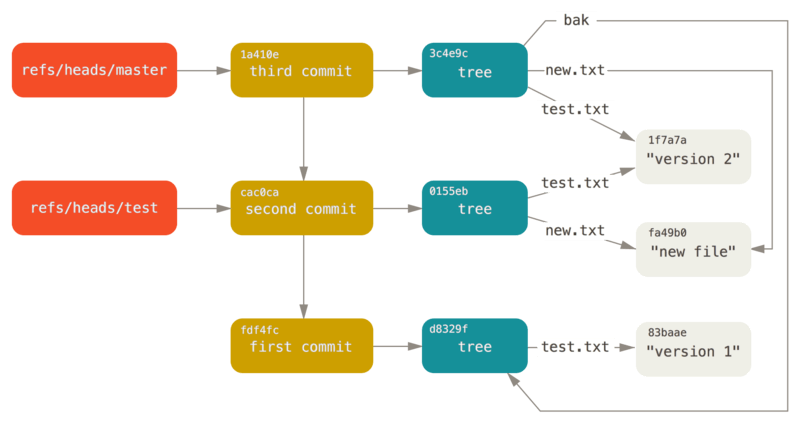
When joining a new project
- On the project page, click the Fork button near the top right to create your own fork. (http://doc.gitlab.com/ce/gitlab-basics/fork-project.html)
- Once the fork is complete, copy the clone URL for your fork. It's in the project header area, above the file list.
- On the command line, git clone that copied URL, then into the newly-created project directory.
- Back on GitHub, go back to the original project page, and copy the clone URL from the project header.
- On the command line, add the repo as a remote source: git remote add main [copied URL]
- You now have two remote sources: main is the original repo and is your fork.
When working on something new
- Check out master: git checkout master
- Create and switch to a new branch: git checkout -b bug_fixes_or_whatever
- Do your work and commit your changes.
When finished with a feature or bug fix
- If you have not yet pushed your branch, make sure the branch is fully up to date with changes that other developers have made:
undefinedundefined - If you have pushed your branch, you should not rebase it, because doing so will require a push --force to overwrite the branch that's on Git; this is generally frowned upon since it will really screw things up if someone else has checked out that branch. Instead, create a new one and rebase that:
undefinedundefinedundefinedundefined - Push your branch to your fork of the repo: git push -u origin bug_fixes_or_whatever (include the -u so you don't need to specify the branch name next time you push)
- On Git, open the project page, in your fork or the original project.
- You'll see a big green button at the top offering to create a new pull request. Click that to start a pull request and review the changes you've made.
- Before or after opening the PR, you can commit more changes and git push them to add them to the PR.
While the PR is in review: if you want to continue working
- Create a new branch from your previous one: git checkout -b more_fixes
- Do NOT push this branch yet. You'll do that later after the previous PR has been merged.
While the PR is in review: if you need to make changes based on code review feedback
- Make sure you have the branch for that PR checked out: git checkout bug_fixes_or_whatever
- Make your changes and commit them
- Push them to your fork: git push
Once a PR has been merged
- Update your local master branch: git checkout master, then git pull main master to get the latest commits from the master branch in the project.
- Push the updates to master to your fork: git push origin master
- Delete your local branch that has been merged: git branch -d bug_fixes_or_whatever. This step isn't strictly required, but you'll quickly find yourself overwhelmed with old branches if you don't keep them tidy.
If you had changes in another new branch while waiting for code review
- Check out your other branch: git checkout more_fixes
- Since that branch was forked from your previous feature branch, you need to rebase it onto master so that your new commits are tacked on after the merge commit: git rebase master
- Now, you can push your new branch: git push -u origin more_fixes
When a project has a new branch, how to add it to my forked repository?
- Make sure you have all changes from the project repository:
git fetch main - Checkout new branch:
git checkout main/newbranch - Push the new branch to your fork:
git push -u origin newbranch